Today we are talking Python, no not those ever-growing snakes found in the everglades, but a very cool programming language you can run from your computer’s terminal.
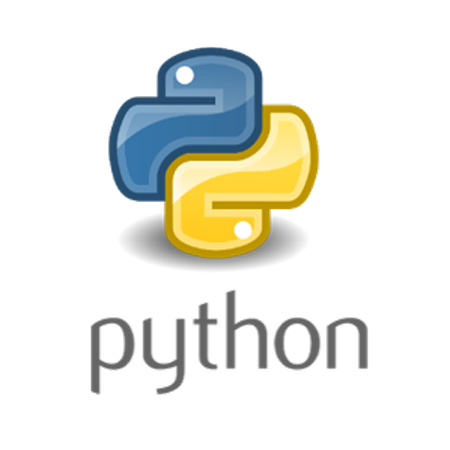
A Little Python History
Python got its start back in 1991 by a programmer named Guido Van Rossum. It’s a high-level general-purpose programming language focused on code readability and ease of use.
The advantage of using Python over say a language like C++ is, in general, there is less coding involved and the code that is created is easier to read.
Python Code
Here is a sample of a Python program that uses BeautifulSoup to look at a website page (https://wwww.indeed.com/) and checks to see if the page has a Title Tag, H1 Tag, and Meta Description.
from bs4 import BeautifulSoup
from urllib.request import Request, urlopen
import urllib.request
url = "https://wwww.indeed.com/"
req = urllib.request.Request(url, headers={'User-Agent': 'Mozilla/5.0'})
html = urllib.request.urlopen(req).read()
soup = BeautifulSoup(html,"html.parser")
#print(soup.prettify())
# ==================================
# Check For Title Tag
if len(soup.title) == 0:
print("No Title Found")
else:
print("Title: ",soup.title)
# ==================================
# Check For H1 tags
rows = soup.find_all('h1') # Find All H1 Tags
x = 0
for row in rows: # Print all occurrences
x = x + 1
print(x,"h1 :",row.get_text())
if x == 0:
print("No h1's Found")
# ==================================
# Check For Meta Description
x = -1
found = 0
metas = soup.find_all("meta")
for meta in metas:
x = x + 1
str1 = str(metas[x])
if str1.find("description") > 1:
print("Meta Descrition: ",str1)
found = 1
if found == 0:
print("No Meta Description Found")
Python Cheat Sheet
Top Things to remember prior to coding in Python
- Case Sensitive – Python is case sensitive and commands must be in lowercase.
- Comments “#“ – to add a comment use the pound sign (“#’). Comments can be made at the beginning of the line or anywhere after a white space, but may not be included within quotes ” “
- Colin “:” – All Python code blocks must use a colon at the beginning of the block. For example, you would add a colon to the end of an if statement to indicate a code block. See the sample code above.
- Block | Indent – To indicate a block of code in Python, you must indent each line of the block by the same whitespace.
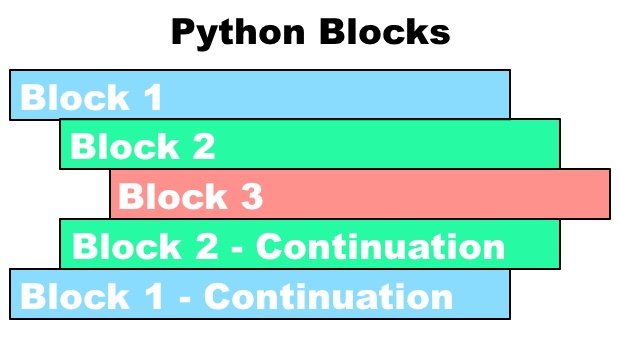
x = -1
found = 0
metas = soup.find_all("meta")
for meta in metas: # Block 1
x = x + 1
str1 = str(metas[x])
if str1.find("description") > 1: # Block 2
print("Meta Descrition: ",str1)
found = 1
if found == 0: # Block 1
print("No Meta Description Found")
Common Operators
- = : Used to assign a value. (Example X = X + 1 or X += 1 or X = -1)
- == : Used for comparison. (Example if x == 1: do something)
- > : Greater Than (Example if x > 1: do something)
- < : Less Than (Example if x < 1: do something)
- != : Not Equal (Example if x != 1: do something)